New study finds social media breaks don’t improve mood, despite the “detox” hype
A new study finds no significant benefits of temporary social media abstinence on well-being.
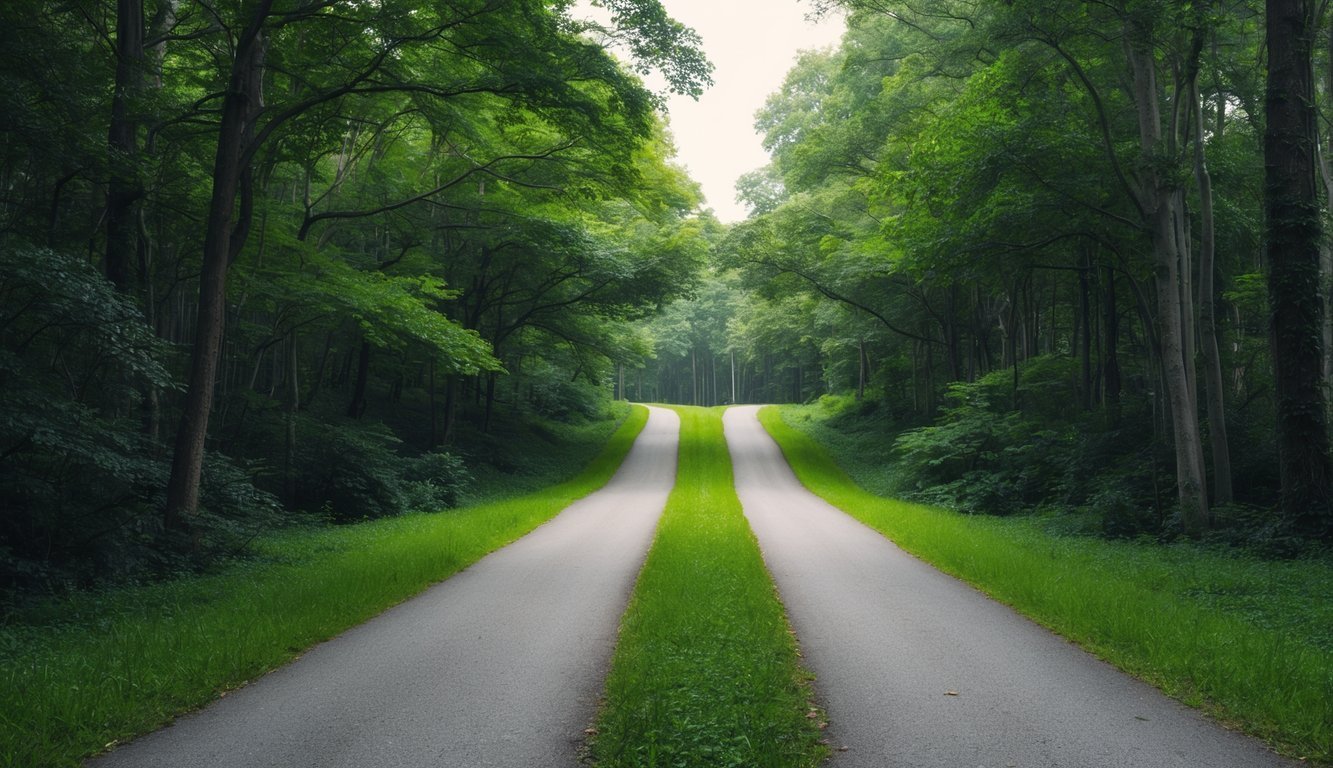
Democrats Dislike Republicans More Than Republicans Dislike Democrats, Study Finds
February 7, 2025
A study of 1,330 Americans reveals strong partisan bias, showing political identity significantly influences social preferences over other identities like race, religion, or income.

Indoor Temperature Significantly Affects Cognitive Function in Seniors
January 22, 2025
Research shows that maintaining indoor temperatures between 68–75 °F is crucial for preventing cognitive decline in older adults, especially amid rising global temperatures.

Physical Inactivity Linked to Higher Anxiety and Depression in Children
January 22, 2025
A study found that physical inactivity in children, especially those with autism or ADHD, is linked to higher anxiety and depression rates, highlighting the need for more support.

Physical Inactivity Linked to Higher Anxiety and Depression in Children
January 22, 2025
A study found that physical inactivity in children, especially those with autism or ADHD, is linked to higher anxiety and depression rates, highlighting the need for more support.
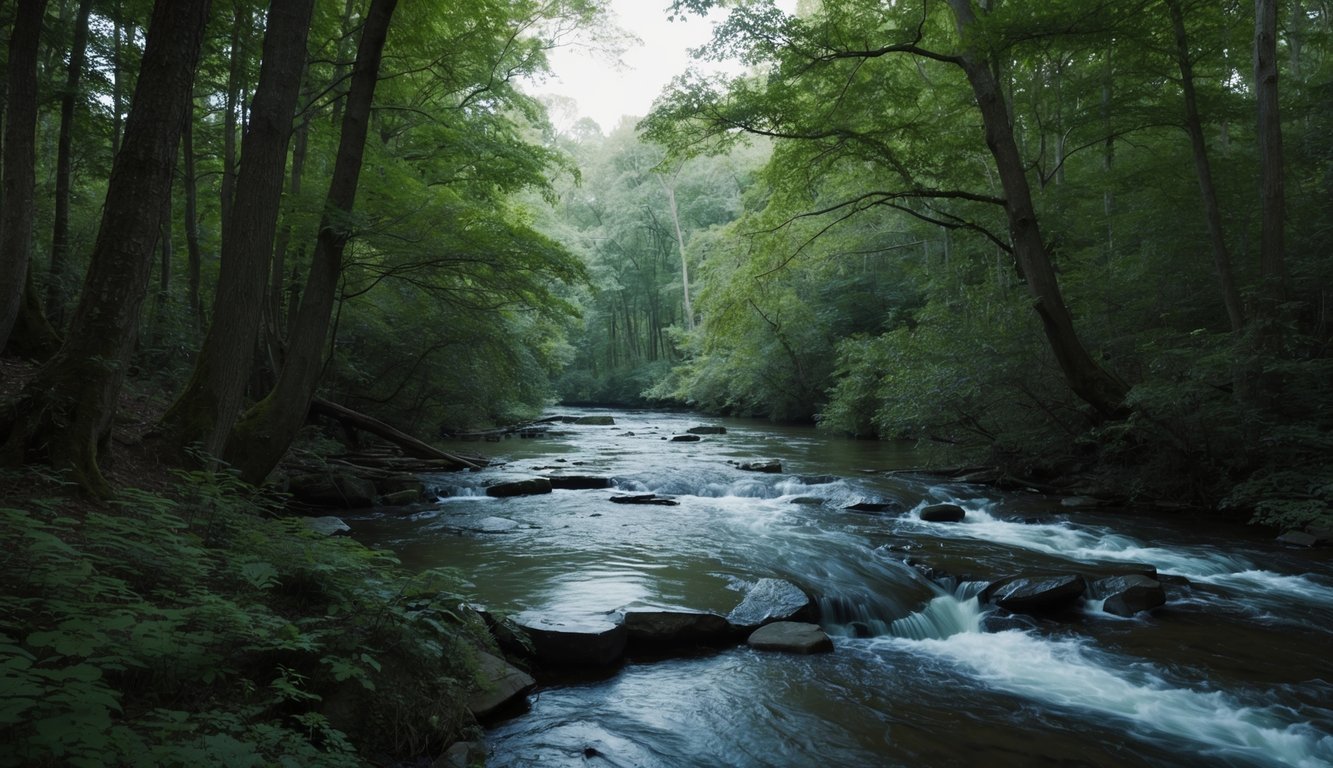
Self-Guided Virtual Reality App Reduces Social Anxiety Symptoms
January 21, 2025
A study found the oVRcome app significantly reduced social anxiety symptoms in users, demonstrating the effectiveness of self-guided virtual reality therapy.
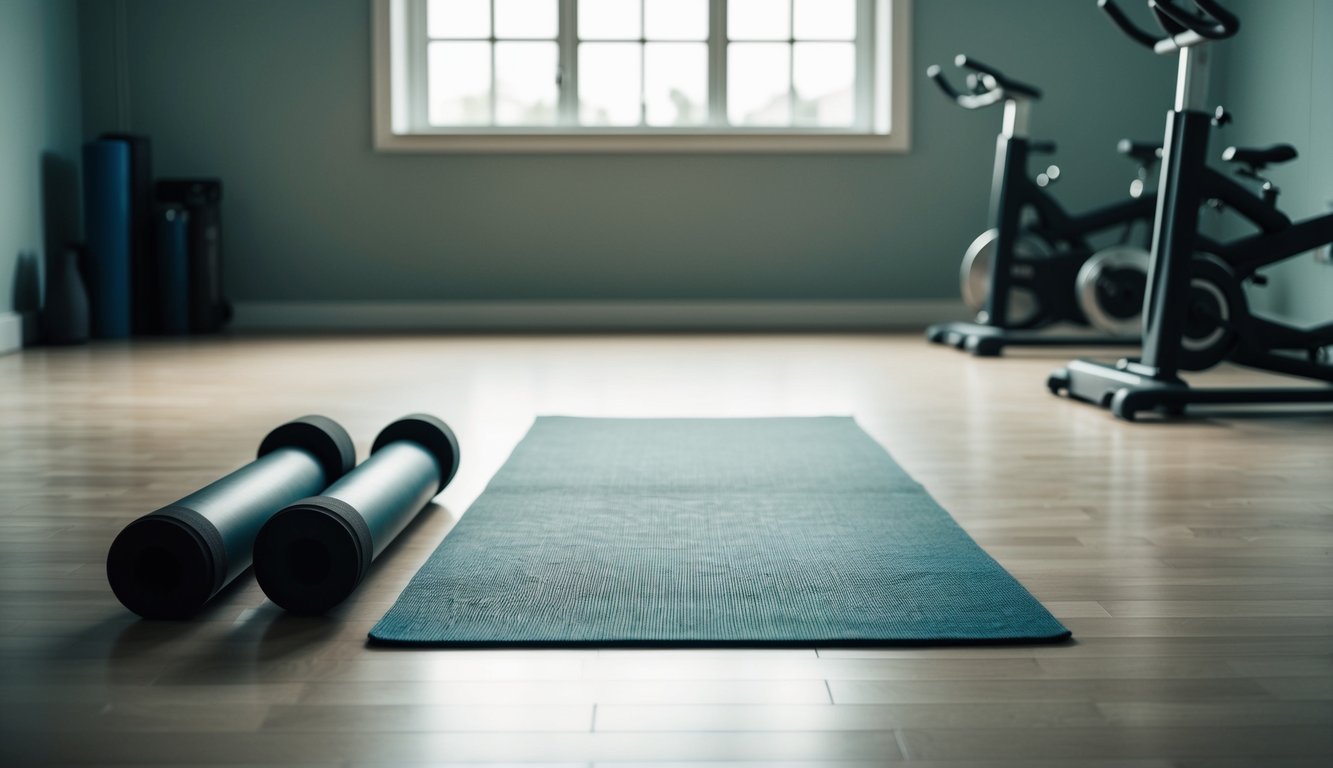
Single Exercise Sessions Boost Cognitive Function in Young Adults
January 21, 2025
A single session of vigorous exercise can enhance cognitive functions like attention and executive skills, offering measurable benefits for brain performance.

Hearing Loss Linked to Increased Parkinson’s Disease Risk, Study Finds
January 21, 2025
A study reveals that a 10 decibel decline in hearing ability may increase the risk of developing Parkinson’s disease by 57%, highlighting a crucial link.
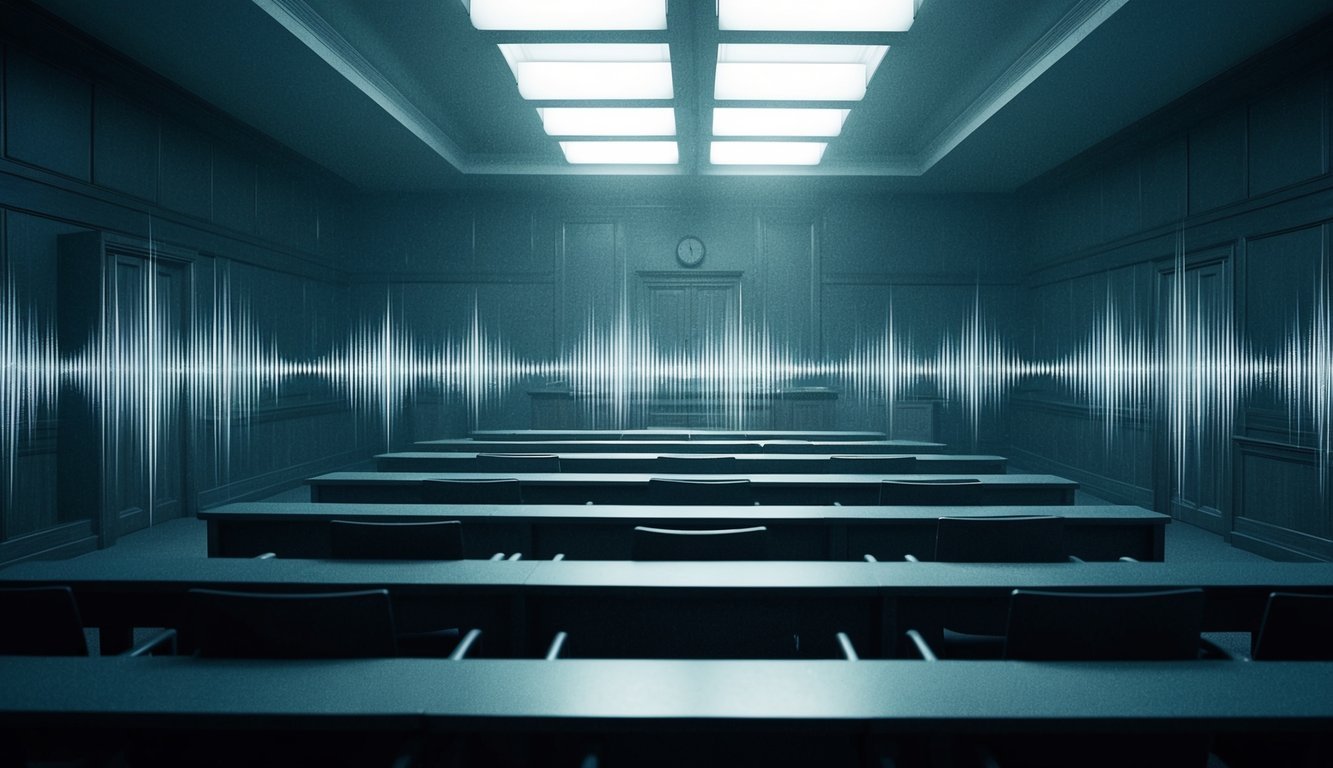
Accents Shape Perceptions of Guilt and Criminality in Justice
January 21, 2025
A study reveals that accents perceived as lower status lead to biased assumptions of criminality, emphasizing the need to address accent-based stereotypes in the justice system.